When comparing Riot vs Ember, the Slant community recommends Riot for most people. In the question“What are the best Angular.js alternatives?” Riot is ranked 6th while Ember is ranked 9th. The most important reason people chose Riot is:
Riot takes the expressions from a DOM tree and stores them in an array. Each of these expressions points to a DOM node. On each cycle these expressions are compared to the values in the DOM. So when a value has changed, Riot automatically updates the corresponding node. This way the operations are kept to a minimal amount and since the expressions can be cached, going through 1000 of them takes less than 1ms.
Specs
Ranked in these QuestionsQuestion Ranking
Pros
Pro Minimal DOM operations
Riot takes the expressions from a DOM tree and stores them in an array. Each of these expressions points to a DOM node. On each cycle these expressions are compared to the values in the DOM. So when a value has changed, Riot automatically updates the corresponding node. This way the operations are kept to a minimal amount and since the expressions can be cached, going through 1000 of them takes less than 1ms.
Pro Lightweight
Riot is made to be used with websites of any kind, so it's built to be easy and lightweight, but still maintaining all the needed features for a UI library. It's only 2.5 KB in size when minified. So it can also be used for mobile web apps without requiring much bandwidth to download.
Pro Components use familiar HTML tags
Riot components use custom tags which are nothing more than familiar HTML tags coupled with JavaScript. This eliminates the need to learn another templating language or syntax. For example:
<todo>
<h3>TODO</h3>
<ul>
<li each={ item, i in items }>{ item }</li>
</ul>
<form onsubmit={ handleSubmit }>
<input>
<button>Add #{ items.length + 1 }</button>
</form>
this.items = []
handleSubmit(e) {
var input = e.target[0]
this.items.push(input.value)
input.value = ''
}
</todo>
Riot tries to separate HTML and JavaScript, while still keeping them inside the component. This way, the HTML can also be neatly mixed with JavaScript expressions.
Pro Supports server side rendering
Riot has support for server side rendering. The views and data are rendered on the server, then those views are sent as HTML to the browser when a user requests them.
This helps with initial loading time and is very useful for SEO purposes because the web app is indexed by search engines same as other static websites that have their HTML on the server.
Pro Easily pluggable with JS/HTML/CSS preprocessors
It is very easy to use your favorite preprocessors with the Riot compiler. Riot comes with CoffeeScript, ES6 (Babel), TypeScript, LiveScript and Jade support. You can also add your own parsers.
Pro Very simple
It makes React look confusing as hell. Nothing against React - It's just that easy to implement!
Pro Scoped CSS available in components
Riot supports scoped CSS inside components for every browser by rewriting stylesheet rules.
Pro Lives well with any other library, framework and usage pattern
Since it's not opinionated, even the scripting can be in anything that can be transpired to JavaScript.
Pro Separation of concerns with RiotControl
RiotControl is inspired by Facebook's Flux Architecture Pattern and it's a simple Central Event Controller/Dispatcher for Riot. It's extremely lightweight (like Riot itself) but unfortunately passes up on some features in favor of performance and simplicity.
RiotControl helps with storing the stater of the application, by passing events from views to stores and vice-versa. Stores can communicate with many views and views can do the same with many stores, this enables to clearly separate concerns and inter-component communication.
Pro Opinionated in terms of application structure
Ember already defines the general application structure and organization for you. This was done to prevent developers from making mistakes which would needlessly over-complicate their application. While it's still possible to go out of these practices forced to developers by the Ember authors, you still have to go out of your way to force them.
Pro Ember-CLI
Ember-CLI is a very useful tool. With just a couple of commands it scaffolds the code, installs dependencies and finally compiles everything itself. It's very useful to quickstart an Ember project.
Pro Uses Handlebars
Ember's preferred templating language is Handlebars. This is mainly because Handlebars is a logic-less templating language and Ember tries to keep it's logic outside the view.
Another reason why Ember benefits from Handlebars is mostly aesthetic as Handlebar's clean syntax makes for easier to read and understand templates.
Finally, Handlebars templates are compiled instead of interpreted, which means that they are much faster to load.
Pro Convention over configuration
Ember follows the philosophy of "convention over configuration" meaning that it already has almost everything configured for you, so you just have to start coding and developing your project right away.
Pro Complete front-end stack
Ember is practically a complete full-stack front-end framework. It comes with it's own asset pipeline, router, services etc...
Pro Completely community based
Pro One of the fastest template rendering engines (new glimmer)
Pro Easy to understand documentation
The Ember Guides are well structured and very well written. The API documentation is also fantastic.
Pro Ember's Object model makes the framework extremely consistent
Most of Ember's components come from the Ember Object Model. It's the basis for views, controllers, models and even the framework itself. This means that the framework is extremely consistent since almost every component shares the same core functionalities and properties since they are all derived from the same object.
Pro Excellent routing
Route handlers for the URLs can see a wide range of possible application states, asynchronous logic in the router makes sure of Promises. And implementing makes sense.
Pro The run loop
It batches bindings and DOM updates to increase performance; if similar tasks are added to a batch, the browser would only need to process them in one single go, as compared to re-computing for each task one at a time.
Pro Excellent API
Ember's API are really easy to understand and work with. It has methods which allow you to harness complicated functionalities in an easy to understand way.
Pro New router has less boilerplate code
Ember's new router need much less boilerplate code that it previously did.
Pro Debugging tool for almost every web browser
Ember also has a debugging tool called Ember Inspector which is used for debugging the client side of your app.
Pro Works great with jQuery
You can use any of jQuery’s features.
Pro Useful bindings
EmberJS provides with an extremely handy feature of advanced bindings. With this you can not only set the path to the binding value in your app but also set in which direction you want the changes to propagate to (oneway
, single
, multiple
etc).
Pro Promises everywhere
Promises represent an eventual state in asynchronous logic. Having promises everywhere (almost) means you could write simple and modular code, using almost any API that Ember provides.
Pro Computed properties
Having custom properties in your templates is itself a huge plus but having custom computed properties is an even greater benefit, since now you can code your custom function as a property and call it from your template. Hence rendering your page exactly according to your needs.
Pro Built-in router
Ember comes with built-in routing capabilities. There's no need to install third-party plugins to be able to use routes.
Pro Auto-updating templates
If you've used handlebars (Ember.js's templating is powered by HandleBars) helper tags in your code (like {{#each}}
) you won't have to worry about updating your template each time you add/remove data from your page, Handlebars will auto update your template for you.
Cons
Con Large library size
At 69Kb gzipped, it is one of the largest JavaScript frameworks. This means Ember might be an overkill to use on simpler projects.
Con Very opinionated
Ember (and many extensions, like Ember Data) force the implementation down specific architectural paths. These paths are what Ember believes is best practice and typically are fine, but not in all cases. This can occasionally lead to fighting with your framework which is never productive.
Con Partly unfriendly community
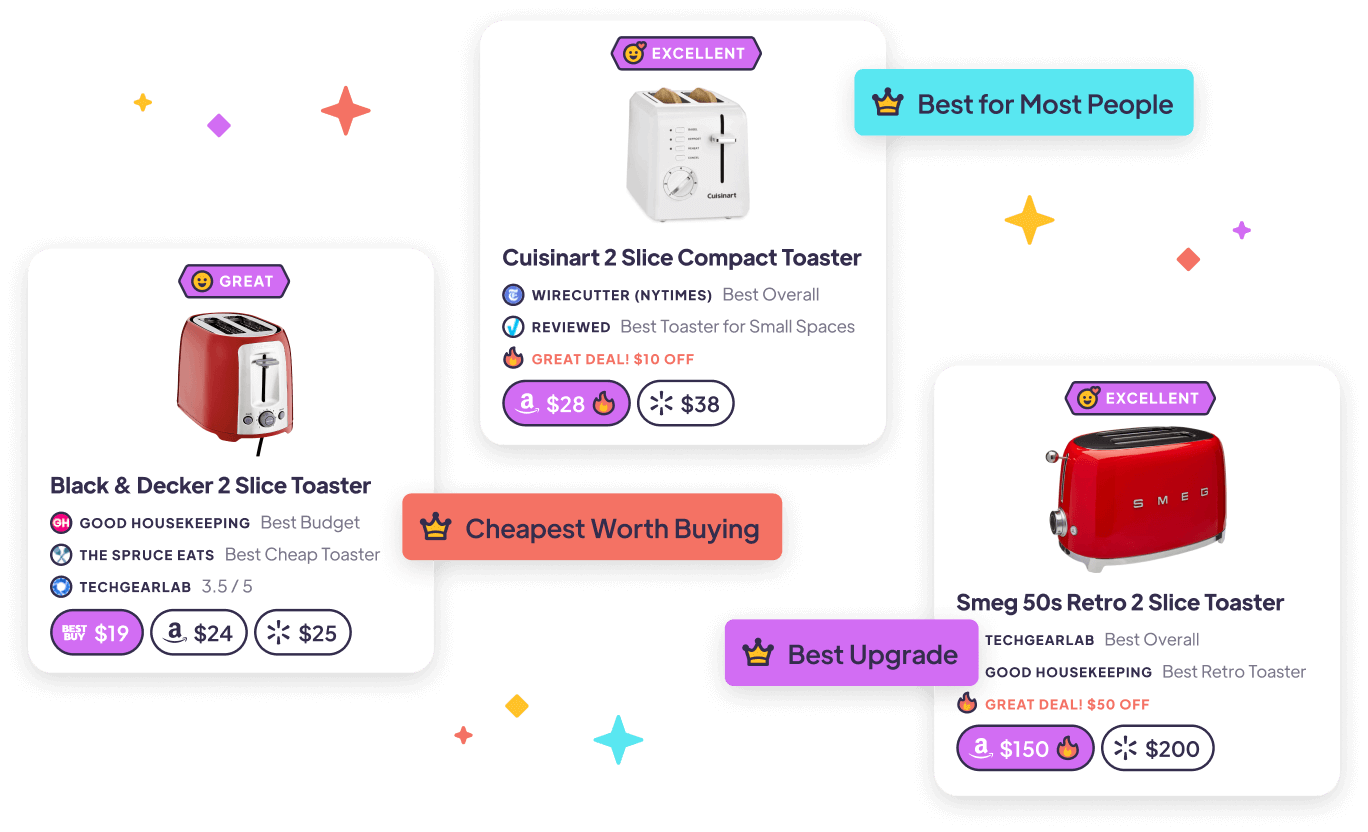