When comparing Ruby vs NPM, the Slant community recommends NPM for most people. In the question“What are the best web design tools?” NPM is ranked 9th while Ruby is ranked 22nd. The most important reason people chose NPM is:
NPM is compatible with any CLI the developer wants to use.
Specs
Ranked in these QuestionsQuestion Ranking
Pros
Pro Widely used
Ruby is one of the most popular languages for developing web sites. As a result, there's an abundant amount of documentation, sample code, and libraries available for learning the language and getting your project up and running. The most popular features are just 'gem install' away. Additionally, it is easier to find Ruby jobs because of this.
Pro Clean syntax
Ruby has a very clean syntax that makes code easier to both read and write than more traditional Object Oriented languages, such as Java. For beginning programmers, this means the focus is on the meaning of the program, where it should be, rather than trying to figure out the meaning of obscure characters.
presidents = ["Ford", "Carter", "Reagan", "Bush1", "Clinton", "Bush2"]
for ss in 0...presidents.length
print ss, ": ", presidents[presidents.length - ss - 1], "\n";
end

Pro A large ecosystem of tools & libraries
Ruby has a large ecosystem of tools and libraries for just about every use. Such as ORMs (Active Record, DatabMapper), Web Application Frameworks(Rails, Sinatra, Volt), Virtualization Orchestration(docker-api, drelict), CLI tools(Thor, Commando), GUI Frameworks(Shoes, FXRuby) and the list goes on. If you can think of it, there is probably a gem for that ( and if not you can create your own and share with the community).
Pro Newbie-friendly community
Pro Essential algorithmic features
The Ruby language is equipped with the necessary features to learn the essence of algorithms.
In online playground environments like ideone.com, measures have been taken to prevent beginners from going astray by restricting the use of external libraries such as Python's NumPy and SymPy.
Even in such constrained Ruby execution environments, the required features for learning algorithms are fully available.
Many of the algorithms that should be learned are documented in the book "Hello Ruby: Adventures in Coding." For example, the cake serving problem in the book leads to topological sorting, which is a graph theory concept useful in project management for creating Gantt charts.
To evaluate the effectiveness of algorithms with a level of complexity comparable to topological sorting,
it is necessary to be able to solve mathematical computation problems up to the high school level easily.
As shown in the table below, using only Ruby's standard library, it is possible to solve high school-level math problems effortlessly.
However, other programming languages may not be able to perform such computations in online playground environments.
To experience the superior performance of algorithms, it is important to challenge oneself by reimplementing good algorithms. Ruby's standard library includes implementations of excellent algorithms. For instance, the algorithm for solving linear equations, which has been widely known since the era of Fortran, is used within the code of SolvingLinearEquations through the "/" operator. Reimplementing code from Ruby's standard library serves as an excellent learning resource with high reusability and efficiency.
The SolvingLinearEquations function mentioned above demonstrates the benefits of duck typing and forced type conversion between objects of different types in arithmetic operations. While Rust also has features like duck typing, the implementation of "forced type conversion" is still far from being realized.
Mathematical Problem Type | Ruby Standard Library | Python Standard Library |
---|---|---|
Long Integer and Fraction | ✓ | ✓ |
Long Integer and Complex Fraction | ✓ | ✖ |
Operations on Matrices with Multiple-Digit Numbers as Coefficients | ✓ | ✖ |
Solution of Integer Coefficient Systems of Equations | ✓ | ✖ |
Solution of Systems of Equations with Long Integer and Complex Fraction Coefficients | ✓ | ✖ |
Solutions of Linear Equations with Real, Fraction, Complex, and Complex Fraction Coefficients | ✓ | ✖ |
# Title: "(1) Cake Serving Procedure Problem"
require 'tsort'
class Hash
include TSort
alias tsort_each_node each_key
def tsort_each_child(node, &block)
fetch(node).each(&block)
end
end
puts 'Tasks'
task_names = {
'A' => 'Arrange the plates.',
'B' => 'Set the spoons.',
'C' => 'Place the birthday cake on the table.',
'D' => 'Spread the tablecloth.'
}
p task_names
puts 'Preceding Tasks'
preceding_tasks = {
'A' => ['D'],
'B' => ['C', 'A'],
'C' => ['A', 'D'],
'D' => []
}
steps = preceding_tasks.strongly_connected_components
puts 'The appropriate steps are as follows:'
steps.each do |task_candidates|
p task_candidates.map { |task| [task, task_names[task]] }
end
p "#(2) Equation Solving Rule"
def SolvingLinearEquations(y, a, b)
x = (y - b) / a
end
p "(2-1) Real Solution", SolvingLinearEquations(1.0, 5, 0.5)
# => 0.1
p "(2-2) Fraction Solution", SolvingLinearEquations(Rational(1, 1), Rational(5, 1), Rational(1, 2))
# => (1/10)
p "(2-3) Imaginary Solution", SolvingLinearEquations(1 + 1i, 5, 1.0 / (2 + 2i))
# => (0.15+0.25i)
p "(2-4) Complex & Fraction Solution", SolvingLinearEquations(Rational(1 + 1i, 1), Rational(5, 1), Rational(1, 2 + 2i))
# => ((3/20)+(1/4)*i)
p "(2-5) Matrix Solution with Large Integers",
SolvingLinearEquations(Matrix[[Rational(1234567890123456789890, 1), Rational(0, 1)]],
Matrix[[Rational(1234567890123456789890, 1), Rational(1234567890123456789890 * 2, 1)],
[Rational(1234567890123456789890, 1), Rational(1234567890123456789890 * 3, 1)]],
Matrix[[Rational(1234567890, 1), Rational(123456789, 1)]] )
# => Matrix[[(3703703670366790122789/1234567890123456789890), (-2469135780244567900789/1234567890123456789890)]]
p "(2-7) Matrix Solution with Large Integers, Complex Numbers, and Fractions",
SolvingLinearEquations(Matrix[[Rational(1234567890123456789890, 1i), Rational(0, 1)]],
Matrix[[Rational(1234567890123456789890, 1), Rational(1234567890123456789890 * 2, 1i)],
[Rational(1234567890123456789890, 1), Rational(1234567890123456789890 * 3, 1i)]],
Matrix[[1234567890, 0 + 1i]] )
# => Matrix[[((-3703703671/1234567890123456789890)-(3/1)*i), ((2469135781/1234567890123456789890)+(2/1)*i)]]
Pro Ruby on Rails
Lays out an easy to follow and opinionated MVC pattern that teaches best practices through necessity.
Pro Test Driven Development, #1
It's the fore-runner and trend setter for TDD.
Pro Hugely object oriented
Object oriented programming is one of the most important concepts in programming.
Pro Meta-programming
Meta-programming provides efficiency and freedom.
Pro No indentation
No indentation increase development efficiency.
Pro Pry
Pro Compatible with any CLI
NPM is compatible with any CLI the developer wants to use.

Pro Plenty of helpful NPM modules/plugins
NPM has a strong community that has developed plenty of libraries and plugins that are useful to developers.
Pro Very concise configuration
NPM scripts require fewer lines of code to run a given task. This is true even when it's for running build processes. Using Unix pipes lots of tasks can be reduced to one-liners.
Pro Does not need any wrapper modules
With other task runners, you need to install wrapper modules for tools you may already have installed. When using NPM that's not necessary, to use the tools you need, just install them directly through NPM.
Pro Part of node.js distribution
Pro You're most likely using NPM already
Pro Uncomplicated package management system
When it works...
Cons
Con Monkeypatching
Requiring a library can change the rules of the language. This is very confusing for beginners.
Con Its ecosystem is limited outside of web development
If you're looking to host, generate, manipulate or secure a website, Ruby is your language. There's also some great support here for infrastructure as code work via Chef. However, it just doesn't have the depth and breadth that Python does. Things like native UI development, high performance math, and embedded / small footprint environments are barely supported at all in Ruby-space.
Con Arcane grammar based on Perl
Ruby is too complicated for beginners:
- arcane Perlisms;
- semi-significant whitespace;
- parentheses are not necessary around method arguments, except for sometimes they are;
- control constructs could be elegantly implemented with block like Smalltalk (Instead they're baked into the grammar.);
- verbose block syntax, unless it happens to be the last argument. (proc lambda).
- There are too many exceptional cases and arcane precedence rules.
Con Meta-programming causes confusion for new developers
The ability for libraries to open classes and augment them leads to confusion for new developers since it is not clear who injected the functionality into some standard class.
In other words, if two modules decide to modify the same function on the same class can introduce a number of issues. Mainly, the order in which the modules are included matters. Since you more or less can't tell what kind of "helper" functions a module might write into any class, or for that matter, where the helper function was included from, you may sometimes wonder why class X can do Y sometimes but not at other times.
Con No docstrings
It's hard to access Ruby's documentation from the REPL (irb), unlike Python, Lisp, and Smalltalk which let you ask functions how to use them, which is a great benefit to the beginner, and which also encourages you to document your program as you code it.
Con More than one way to do it
A problem inspired by Perl. The core API interfaces are bloated. There's at least four different ways to define methods. More is not always better. Sometimes it's just more.
Con Does not teach you about data types
Since Ruby is a dynamically typed language, you don't have to learn about data types if you start using Ruby as your first language. Data types being one of the most important concepts in programming. This also will cause trouble in the long run when you will have to (inevitably) learn and work with a statically typed language because you will be forced to learn the type system from scratch.
Con Dynamic type system
Majority of bugs could be resolved with types.
Con Viewed as a web development language
Despite its flexibility and performance, Ruby is often seen as being unsuitable for other tasks by those who are not familiar with it. As such, a lot of discussion about it centers around Rails, which is not at all relevant if you're using Ruby for something else, such as game development.
Con Focus on Object-Oriented Programming (OOP)
Focussing on OOP in a beginner stage is an easy and popular plan, but not the best one.
Con Custom tasks require additional keyword 'run'
Only a few standard tasks support being executed without the run
keyword (e.g., npm start
vs npm run customtask
)
Con Not a build system, only a task runner
It is supposed to be used for running gulp, webpack or whatever. But it is not supposed to be used as a build system.
Con Passing parameters is awkward
In order to pass additional parameters to npm you must add them after --
(e.g., npm run build -- --custom='foo'
).
Con Badly documented
Less than bare minimum official documentation leaves users in the dark without taking often expensive external courses. Even the --help text has unpluggable gaps. One official source notes the documentation isn't adequate yet nothing has been done to fix this.
Con Lot of issues with authentication and random node problems
Unable to recover from common depencies conflicts consistantly. Error messages are not always helpful to debugging. Doesn't account well for users with different versions of node.
Con Does not run well with Windows
Since a lot of projects that use NPM as a build tool most of the time make use of Bash scripts as well. This means that open source projects that run the command npm run
may run into issues when used in a Windows environment.
Con Doesn't allow you to create build process with complicated logic on its own
In complex heterogeneous app you will quickly migrate to gulp, webpack or whatever leaving to NPM only simple task running responsibility.
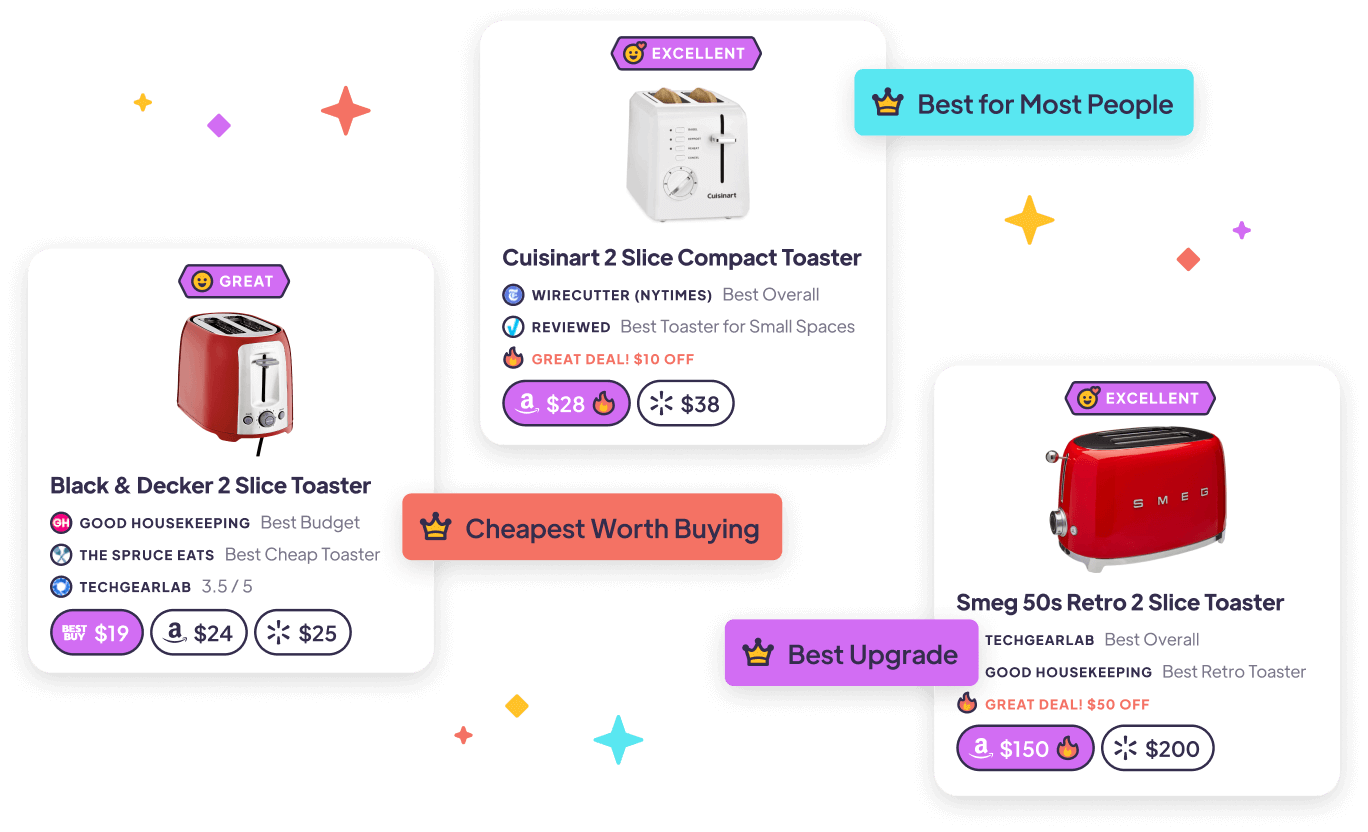